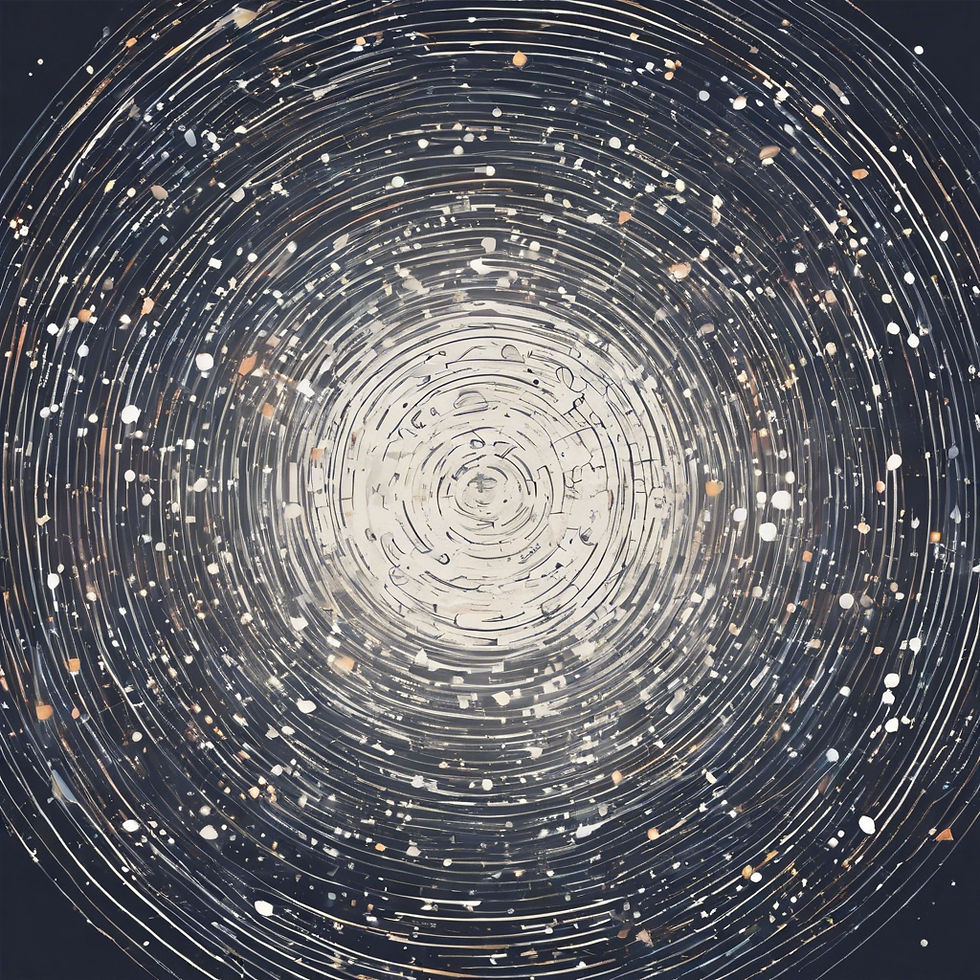
1. Comparison-Based Sorting Algorithms
Bubble Sort: Repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. It's simple but inefficient for large datasets, with a worst-case time complexity of O(n^2).
Selection Sort: Finds the minimum element from the unsorted part of the list and moves it to the beginning. It also has a worst-case time complexity of O(n^2) but performs fewer swaps than bubble sort.
Insertion Sort: Builds the sorted array one item at a time by repeatedly picking the next item and inserting it into the correct position in the already-sorted section. Time complexity is O(n^2) in the worst case but O(n) in the best case if the data is already sorted.
Merge Sort: Divides the array into halves, sorts each half, and then merges the sorted halves. It has a time complexity of O(n log n) and is efficient for large datasets.
Quick Sort: Chooses a 'pivot' element, partitions the array around the pivot, and recursively sorts the partitions. Its average-case time complexity is O(n log n), but it can degrade to O(n^2) in the worst case.
Heap Sort: Builds a heap from the array and then repeatedly extracts the maximum element from the heap to build the sorted array. It has a time complexity of O(n log n) and is useful when constant space usage is important.
Shell Sort: An extension of insertion sort that allows the exchange of items that are far apart. The time complexity can vary depending on the gap sequence used, with average cases around O(n log n) to O(n^(3/2)).
2. Non-Comparison-Based Sorting Algorithms
Counting Sort: Counts the occurrences of each distinct element and uses this count to determine the position of each element in the sorted array. It’s efficient with time complexity O(n + k) where k is the range of the input.
Radix Sort: Sorts numbers digit by digit, starting from the least significant digit to the most significant. It uses counting sort as a subroutine. Time complexity is O(n * k), where k is the number of digits.
Bucket Sort: Divides the elements into several buckets and then sorts each bucket individually, usually with another sorting algorithm. It performs well when the input is uniformly distributed, with time complexity O(n + k).
3. Adaptive and Hybrid Sorting Algorithms
Timsort: A hybrid sorting algorithm derived from merge sort and insertion sort, designed to perform well on many kinds of real-world data. It has a time complexity of O(n log n) and is used in Python’s built-in sort and Java’s Arrays.sort() for objects.
IntroSort: A hybrid sorting algorithm that begins with quicksort and switches to heapsort when the recursion depth exceeds a level based on the number of elements being sorted. It’s used in the C++ Standard Library’s std::sort.
Conclusion: Each algorithm has its own best-use scenarios, and the choice of algorithm can depend on the size of the dataset, the nature of the data, and other constraints such as memory usage.
Comments